What is the Symbol Language?

The Symbol language is a script language, a bit like python, which is used as the main language to write commands for the OnScale Flex Solver
The engineers who developed that language 30+ years ago needed to have a script language, but there was none at the time! So they decided to develop this “Symbol” Language which is based on FORTRAN.
What can you do with the Symbol Language?
The Symbol Language has plenty of capabilities, such as:
- Define variables for inputs to commands
- Math functions (sin, cos, nint, max)
- Loops, if statements, procedures
- IO functions (Read/Write Files)
- Access variables in Memory
- Execute external software
- Etc…
Introduction to Symbol – Video
What I will teach you in this video:
- Symbol variables and Expressions
- Loops
- Procedures
- Printing more info for debug
- Writing messages to the console and to files
- Doing process calls
- Extracting value from an array and printing it to text
A Few Rules to Define Symbol Variables
Numeric variables are implicitly typed according to the default Fortran convention
i.e: In older versions of Fortran, e.g. 77, variables did not need to be declared. If the name starts with I, J, K, L, M, or N then it would be assumed to be an INTEGER. Otherwise, it would be assumed to be REAL (float).
‘symb’ command is used to define variables
- Integer numeric variables must have names beginning with the letters I-N
- Real numeric variables must have names beginning with the letters A-H, O-Z

A Few Rules to Define Symbol Variables
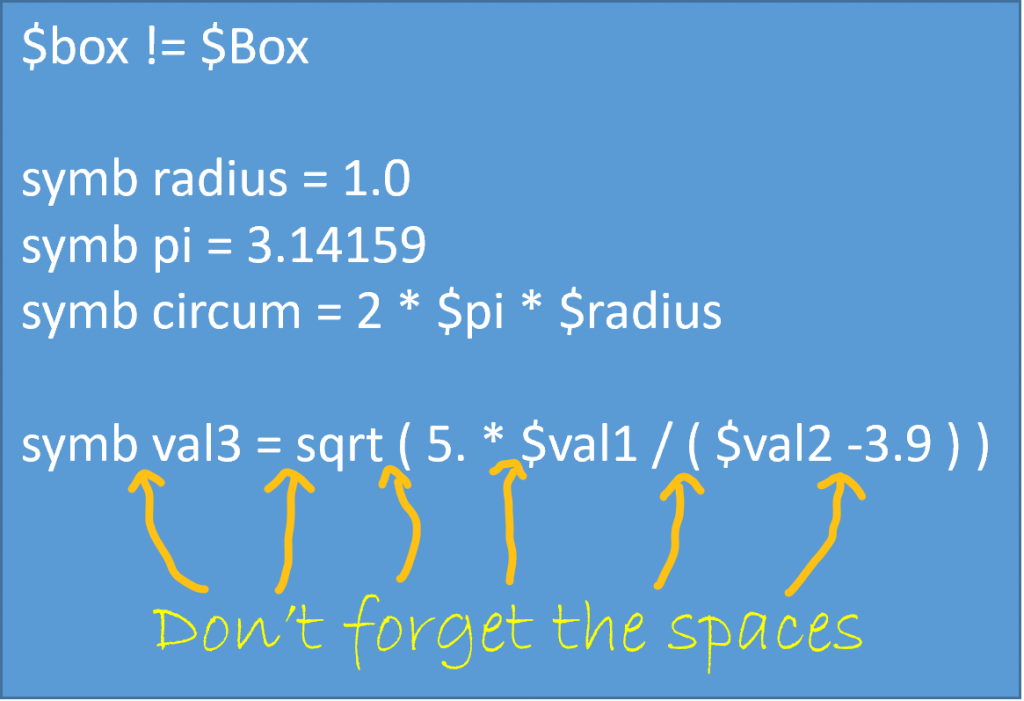
Variables are case sensitive, so remember to pay attention to the capital letters!
You have to use the dollar sign $ before a variable name to represent the value stored into the variable
All symbol entries must be blank delimited. This means that you must have spaces present between all numbers, variables, operand of functions and parentheses.
Quick Test and Variable Manager
After you run your code at least one time, your variables will be saved into the “Variable Manager”.
This is very useful to know if you want to rapidly check the value associated to a specific variable of your model!

Writing a message to the console
Let’s talk now about writing some text to the console (or into a text file in the next section).
Basic Syntax:
Symb #msg 1
Hello world!
Symb #msg 2
Mesh Size:
box = $box
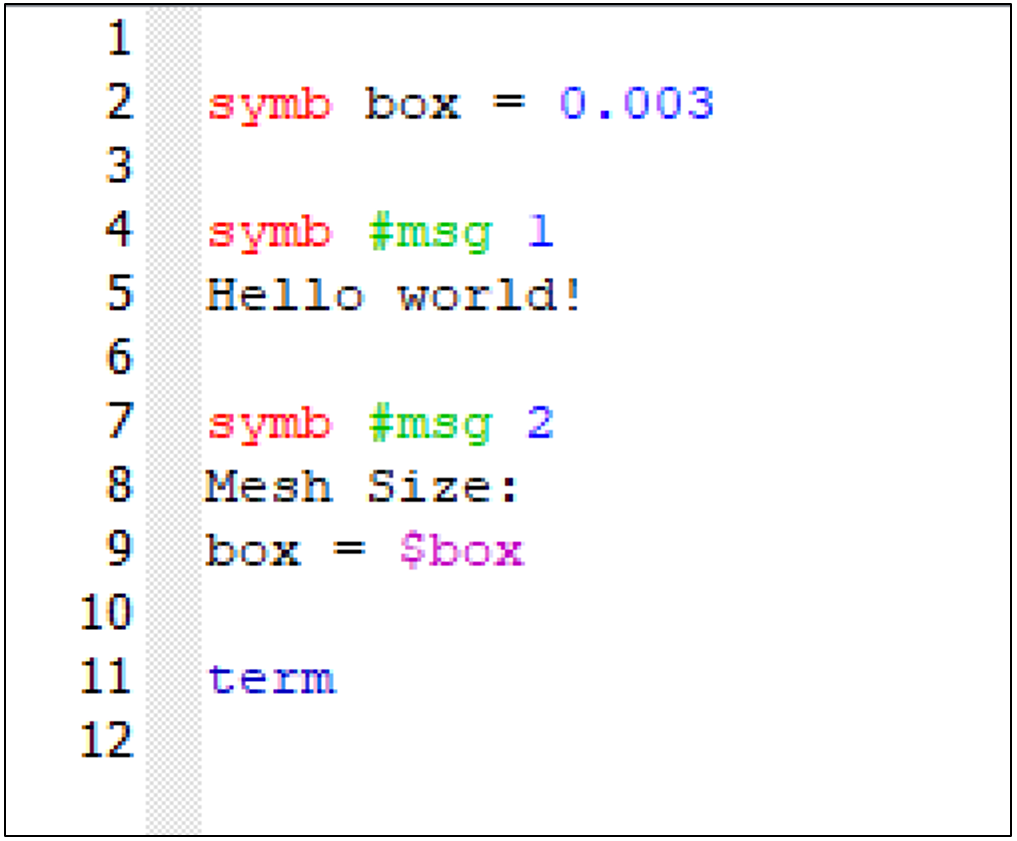
The integer after the #msg tag refers to the number of lines that will be sent to the console. you can put some variables in it by calling variable which have been already declared in your model with a dollar sign $.
Writing a message to a file
You can write to a file by adding “>” and the name of the file
Using “>>” will append the text to an existing file

Writing a Loop in Symbol
Basic Syntax:
do LoopName i 1 10 1
(instructions)
end$ LoopName
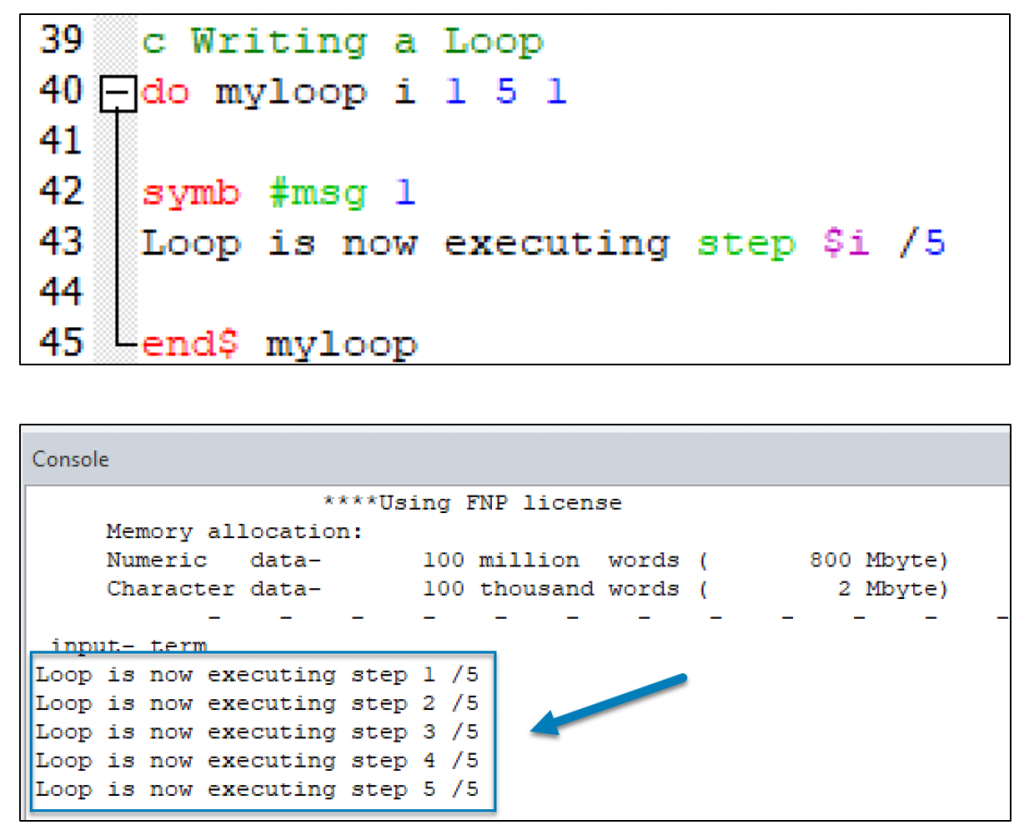
i is the name of an integer Symbol variable which will be defined and incremented during the execution of the do loop
LoopName is the name of the loop
If – Elseif conditional flows
Basic Syntax:
if ( datum1 op datum2 ) then
(instructions)
elseif ( datum1 op datum2 ) then
(instructions)
endif
datum1 and datum2 can be either numeric or character data.
op is any of the conditional operators: eq, ne, lt, le, gt and ge whose meanings correspond to their usage in Fortran.

Using Procedures (Custom functions)
A procedure is exactly like a custom function
proc warmGreetings save
symb #msg 1
This is the step $I/5 of calculation
end$ proc
proc warmGreetings 5
term
You need to define it first and then you can execute it as much as you need!

Using Mathematical Operators
The SYMB statement can also take the form:
symb vname = <expression>
The <expression> may be composed of the following mathematical operations:
COS, SIN, TAN, EXP, ALOG, ALOG10, ACOS, ASIN, ATAN, ATAN2, SQRT, ABS, SIGN, INT, NINT, MAX, MIN, **, *, /, +, and -.
Parentheses may be used to control the sequence in which the expression is evaluated (innermost parentheses evaluated first). Within parentheses, the evaluation is done from left to right for each operator listed above in the order listed.
Symbol Built-in functions
Many functions are available by default:
symb #get { ilength } charlen string
symb #get { numsectotal numsec nummin numhr numday } iwallclock
symb #get { ii ij ik rdist } clsnode (grid) x y z ibegin iend jbegin jend & kbegin kend
Those are just 3 of the available functions, much more exist, check the documentation for the details!
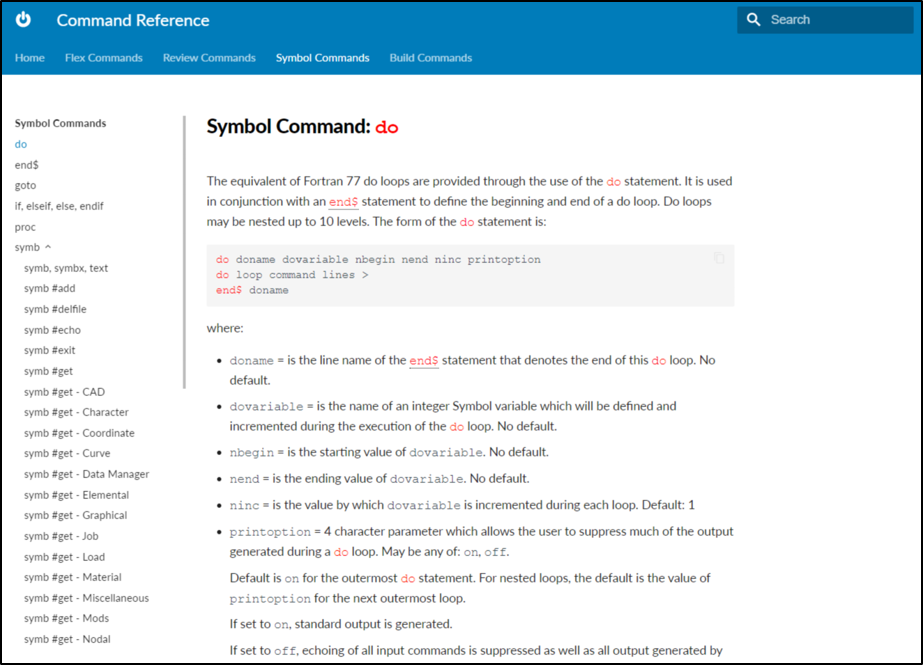
That’s all for today, Hope this is useful!
Don’t hesitate to leave some questions in the comment section if you have some!
–Cyprien “Introducing you to Symbol” Rusu
Leave a Reply