I talked about how to use Salome to create geometry with Python Previously…
Time to go a bit deeper!
Let’s have a look at how to use Python to generate a Concrete Model with Aggregates ;-)
Why would I want to generate such a model?
Reason 1- This methodology can be used for any type of material or model which has some repetition baked into it, so it’s a really useful tutorial.
Reason 2- Such model can be used for certain very specific types of simulations which require an exact modeling of the concrete structure. Think about the case in which we would like to perform an ultrasonic inspection of a concrete block for example to spot some defects
You will learn:
- How to generate a Concrete Block
- How to use Python to generate the aggregates in it with a loop
This is the final python code I used to generate the concrete with aggregate model:
import sys
import salome
salome.salome_init()
theStudy = salome.myStudy
import salome_notebook
notebook = salome_notebook.NoteBook(theStudy)
# Change that to the directory where your salome is installed
sys.path.insert( 0, r'C:/Program Files/sm-2018-w64-0-3/WORK')
###
### GEOM component
###
import GEOM
from salome.geom import geomBuilder
import math
import SALOMEDS
import random
geompy = geomBuilder.New(theStudy)
O = geompy.MakeVertex(0, 0, 0)
OX = geompy.MakeVectorDXDYDZ(1, 0, 0)
OY = geompy.MakeVectorDXDYDZ(0, 1, 0)
OZ = geompy.MakeVectorDXDYDZ(0, 0, 1)
Box_1 = geompy.MakeBoxDXDYDZ(200, 200, 200)
Spheres = []
for i in range(200):
X = random.uniform(0,200)
Y = random.uniform(0,200)
Z = random.uniform(0,200)
r = random.uniform(0,6)
Vertex_1 = geompy.MakeVertex(X, Y, Z)
Sphere_1 = geompy.MakeSpherePntR(Vertex_1, r)
Spheres.append(Sphere_1)
Partition_1 = geompy.MakePartition([Box_1], Spheres, [], [], geompy.ShapeType["SOLID"], 0, [], 0)
geompy.addToStudy( O, 'O' )
geompy.addToStudy( OX, 'OX' )
geompy.addToStudy( OY, 'OY' )
geompy.addToStudy( OZ, 'OZ' )
geompy.addToStudy( Box_1, 'Box_1' )
geompy.addToStudy( Partition_1, 'Partition_1' )
if salome.sg.hasDesktop():
salome.sg.updateObjBrowser(True)
If it was useful, please give a like to the video and help me to spread the knowledge, Thanks!
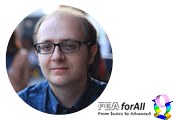
Cyprien “Making Concrete Tutorials” Rusu
Leave a Reply